This post was most recently updated on July 31st, 2024
Execute selenium script in Brave browser
To download and install selenium configuration please refer link:
1.Download and install brave browser in system:
->Go to Brave browser site.
->Download Brave Browser according to your system configuration.
As I have 64 bit window machine ,I will download brave browser for 64 bit.
->Install the brave browser in the system
Search for brave browser in google or download it from here
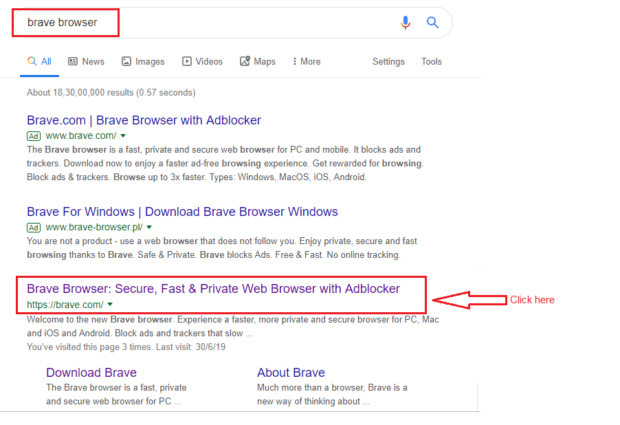
Click on Download button
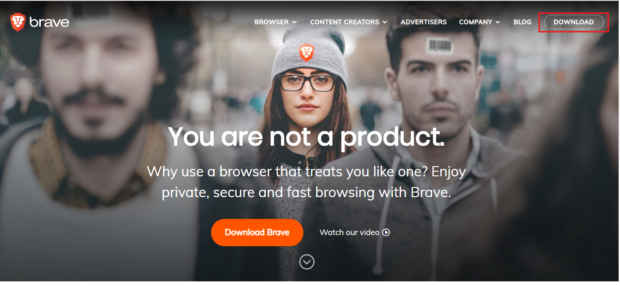
Select link based on your system configuration
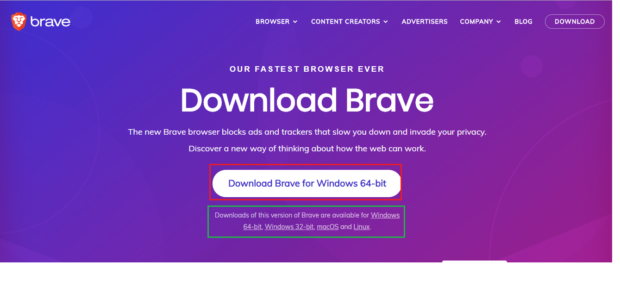
Install the brave browser in the machine.
2.Check that your chrome driver version is compatible with your brave browser version.
Check chrome driver version
-Click on chrome driver application file
-Check the chrome driver version.
Check brave browser version
-Go to settings of your brave browser.
-Click on “About brave”
-Check the chromium version of brave browser
If chrome driver version is not compatible with your brave browser version then update the chrome driver version.
Selenium code to open brave browser using selenium
System.setProperty(“webdriver.chrome.driver”,”path of chrome driver”);
ChromeOptions options=new ChromeOptions();
//Set the path of brave browser
options.setBinary(“C:\\Program Files (x86)\\BraveSoftware\\Brave-Browser\\Application\\brave.exe”);
WebDriver driver=new ChromeDriver(options);
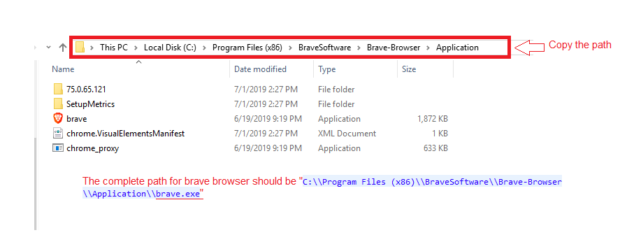
Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
package selenium; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; public class BraveBrowser { public static void main(String[] args) { //Set the path of chrome driver System.setProperty("webdriver.chrome.driver","D:\\selenium\\chromedriver_win32\\chromedriver.exe"); //Create object of ChromeOptions class ChromeOptions options=new ChromeOptions(); //Set the path of brave browser options.setBinary("C:\\Program Files (x86)\\BraveSoftware\\Brave-Browser\\Application\\brave.exe"); WebDriver driver=new ChromeDriver(options); driver.manage().window().maximize(); driver.get("https://www.google.com"); driver.findElement(By.xpath("//a[text()='Gmail']")).click(); driver.navigate().back(); WebElement search=driver.findElement(By.xpath("//input[@name='q']")); search.sendKeys("selenium" ,Keys.ENTER); } } |