This post was most recently updated on July 31st, 2024
List is the most commonly used collection in Python. It is used to store list of variables which may have different data types. It is also useful to implement stacks and queues data structures. Variables can be dynamically stored using Lists.
Following are some features of list
1. Lists are written with square brackets.
2. Functions, modules used in the script can also be stored in List
3. Duplicate records are allowed in list.
4. Created list can be updated as and when required.
Following operations can be performed on List:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
#Creating List List1 = [] print("Created List: ",List1) #Adding records to List List1.append(1) List1.append(4) List1.append('Mundrisoft') List1.append(5) List1 = [1,4,'Mundrisoft',5] print("List after adding records: ",List1) #Adding record to specific index List1.insert(2,'Pune') print("Updated List: ",List1) #Creating a list inside a list List2 = ['Country', 1, 'State'] List1.append(List2) print("Nested Lists ",List1) #Adding multiple records at the end of the list List1.extend([6,7,8,'City']) print("Multiple records added: ",List1) #Accessing 2 element from the list print("Element at index 2: ",List1[2]) #Accessing element using negative index. Print second Last element print("Second last element of List: ",List1[-2]) #Deleting element from List del List1[3]; print("After deleting element from index 2: ",List1) #Remove element from List List1.pop() print("After popping element: ",List1) #Remove element from specific location List1.pop(3) print("After popping element from index 3: ",List1) |
Output:
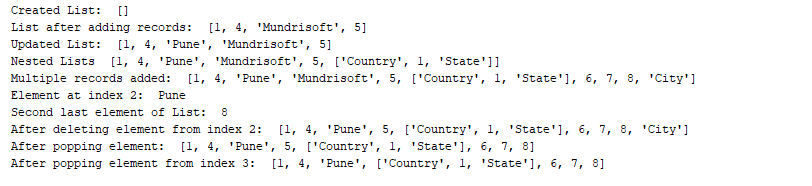