How to read write data in notepad file using selenium?
Below is Selenium code to perform read and write operation in notepad file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 |
package lib.notepad; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.File; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.util.ArrayList; import java.util.List; public class NotepadReadWrite { public static void printText(String filePath) throws IOException { List<String> lines = readTextFile(filePath); System.out.println("\n Print notepad file_________________"); for (String line : lines) { System.out.println(line); } System.out.println("___print operation completed___"); } public static void createNotepad(String filePath) throws IOException { File fp = new File(filePath); if (!fp.exists()) { System.out.println("Create notepad file__________________"); if (!fp.getParentFile().exists()) { fp.getParentFile().mkdirs(); } fp.createNewFile(); } } public static List<String> readTextFile(String filePath) throws IOException { // use BufferedReader to read notepad file line by line FileReader fReader = null; BufferedReader bfrReader = null; // Store lines in a List<String> lines = new ArrayList<>(); File fp = new File(filePath); if (fp.exists()) { System.out.println("\n Reading notepad file________________"); fReader = new FileReader(fp); bfrReader = new BufferedReader(fReader); String line = null; do { // Read one line and store it in String variable line = bfrReader.readLine(); if (line != null) { lines.add(line); } } while (line != null); bfrReader.close(); fReader.close(); } else { System.out.println("File does not exist"); } return lines; } public static void appendLine(String filePath, String line) throws IOException { // read old notepad file and store lines List<String> lines = readTextFile(filePath); // add new line to the list lines.add(line); FileWriter fWriter; BufferedWriter bWriter; File fp = new File(filePath); if (fp.exists()) { System.out.println("Appending to the notepad file_________________"); fWriter = new FileWriter(fp); bWriter = new BufferedWriter(fWriter); // write to notepad file for (String oneLine : lines) { bWriter.append(oneLine); bWriter.newLine(); } bWriter.close(); fWriter.close(); } else { System.out.println("File does not exist"); } } } |
Main class to execute read and write function from above class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
package lib.notepad; import java.io.IOException; import java.util.Date; public class NotepadMain { public static void main(String[] args) throws IOException { // below folder structure and text file not available in system String newNotpadFilePath = "D:\\NewFolder\\TextFile.txt"; // Create new text file NotepadReadWrite.createNotepad(newNotpadFilePath); // Print : Nothing will printed NotepadReadWrite.printText(newNotpadFilePath); // Append data in text file NotepadReadWrite.appendLine(newNotpadFilePath, "Add message\t" + new Date().toString()); // Print that data NotepadReadWrite.printText(newNotpadFilePath); } } |
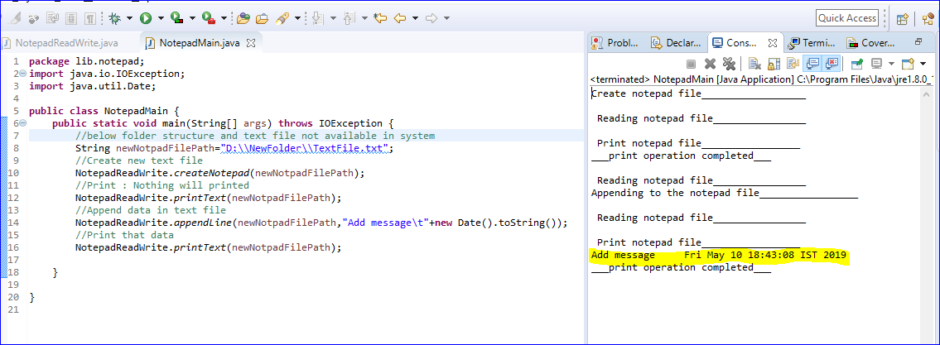