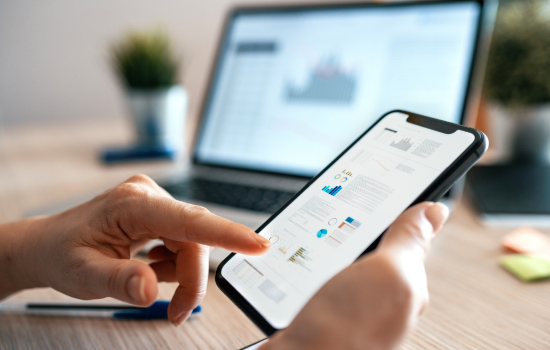
Effective Strategies to Prevent App Crashes and Improve Debugging Mechanisms
Introduction
Mobile applications are integral to modern digital experiences, but frequent crashes can frustrate users, lead to negative reviews, and impact revenue. Crashes often stem from inadequate testing, unhandled exceptions, memory leaks, or compatibility issues across devices and operating systems. To ensure a seamless user experience, developers must adopt robust strategies for testing, performance optimization, and debugging.
This guide explores key strategies for preventing app crashes and enhancing debugging mechanisms. From functionality testing and memory management to real-time testing and network resilience, we cover essential steps for building a stable and high-performing mobile application.
Table of Contents
1. Functionality Testing
Ensure all features work as expected from the user’s perspective. Key scenarios include:
- Textbox validation
- Page navigation consistency
- Data input/output accuracy
Expert Tip: Automate functionality tests to speed up validation and reduce human errors.
2. Regression Testing
Continuously test new updates to identify potential bugs introduced by changes. Maintain stability by:
- Automating regression tests for faster cycles
- Testing both functional and non-functional aspects
Expert Tip: Use CI/CD pipelines to integrate automated regression testing seamlessly.
3. Performance Optimization
Test the app’s responsiveness under different conditions:
- High user load testing
- Handling interruptions (calls, SMS, background apps)
- Evaluating download speed and file size efficiency
Expert Tip: Use profiling tools like Android Profiler and Xcode Instruments to identify bottlenecks.
4. Error Handling and Exception Management
Ensure the app gracefully handles errors such as:
- Incorrect user inputs
- Unexpected API responses
- Temporary network failures
- Memory allocation errors
Expert Tip: Implement logging frameworks like Logcat or Sentry for better error tracking.
5. UI Response Time Improvement
- Measure and optimize response times based on network latency and OS behavior
- Improve rendering efficiency for smoother interactions
Expert Tip: Optimize animations and minimize redundant UI redraws to enhance user experience.
6. App Size and Storage Optimization
- Minimize app size without sacrificing functionality
- Optimize caching and avoid excessive storage use
- Test performance under low-storage conditions
Expert Tip: Use ProGuard or R8 for code shrinking and optimization.
7. Third-Party Integrations Testing
Ensure seamless integration with:
- Built-in device functionalities (e.g., GPS, camera, contacts)
- Third-party services (e.g., analytics, crash reporting, payment gateways)
Expert Tip: Always test third-party SDK updates before rolling them out in production.
8. Real-Time Scenario Testing
- Test under various network conditions, time zones, and GPS locations
- Simulate real-world interruptions such as SMS notifications and low battery alerts
Expert Tip: Use cloud-based testing tools to cover multiple geolocations and devices.
9. OS Upgrade Monitoring
- Ensure compatibility with new OS updates
- Test the app against beta versions of OS releases
Expert Tip: Participate in OS beta programs to stay ahead of potential compatibility issues.
10. Network Resilience and Connectivity Handling
- Implement robust error handling for network failures
- Use retry mechanisms and caching strategies for interrupted requests
Expert Tip: Implement exponential backoff strategies to handle intermittent connectivity issues.
11. Compatibility Testing Across Devices
- Test across various OS versions, screen sizes, and hardware specifications
- Maintain a test list of commonly used devices
Expert Tip: Use automated cross-device testing platforms like BrowserStack.
12. Memory Management Best Practices
- Detect and resolve memory leaks using profiling tools (e.g., Android Profiler, Xcode Instruments)
- Optimize cache usage to prevent excessive memory consumption
Expert Tip: Use weak references where applicable to prevent memory leaks.
13. Database Connection Stability
- Ensure proper handling of concurrent database access
- Implement offline data synchronization strategies
Expert Tip: Optimize database queries and indexing to improve efficiency.
14. Background Process Management
- Test how the app behaves when running in the background or sleep mode
- Optimize background activities to avoid excessive CPU usage
Expert Tip: Use WorkManager (Android) or BackgroundTasks (iOS) for optimized background processing.
15. Battery Consumption Optimization
- Identify and reduce energy-intensive background activities
- Optimize resource usage without compromising functionality
Expert Tip: Profile power consumption using Battery Historian (Android) or Instruments (iOS).
16. Regular Updates and Maintenance
- Release periodic updates to fix bugs and enhance stability
- Keep up with platform updates and evolving best practices
Expert Tip: Monitor user feedback to prioritize bug fixes and improvements.
17. Increasing Automated Test Coverage
- Expand test scripts to cover critical workflows
- Use AI-driven test automation tools to improve efficiency
Expert Tip: Leverage AI-based testing tools like Appium and Test.ai for automated UI testing.
18. Advanced Crash Analysis and Debugging
Adding Custom Keys
Custom keys help capture the app’s state leading up to a crash. Useful keys include:
- Network Connection State: WiFi, 4G, 3G, No Internet
- Locale: User’s region settings (e.g., en_US, es_ES)
- Density Qualifier: Device screen density
- Background Crash: Identifies crashes occurring when the app is in the background
Adding Custom Events
Track specific user interactions and system events before a crash occurs. Examples include:
- API request failures
- UI interaction patterns before a freeze
- Background-to-foreground transitions
Debugging App Crash Logs
Sample Crash Logs:
Common app crash errors include:
org.company.pfff.data.DataHelper.getEnabledCountries
org.company.pfff.data.DataHelper.getBrowsableCountries
org.company.pfff.data.DataHelper.getUpdatingCountriesCount
org.company.pfff.adapter.CountryListAdapter.getView
android.text.SpannableStringBuilder.checkRange
org.company.pfff.model.Factsheet.getImagesForSection
Root Cause Analysis:
Issues 1-4 arise due to missing database columns (e.g., “country.totalcountofdatasheets”).
If a database is modified (e.g., adding a column/table), the app may not auto-update when installed over a previous version.
Solution: Implement database migration strategies to retain user data.
Issue 5 (android.text.SpannableStringBuilder.checkRange):
Likely due to accessibility features.
Steps to reproduce:
- Enable TalkBack on the device.
- Open the app.
- Download country data.
- Click on “Browse by Problem.”
By implementing these strategies, developers can significantly reduce app crashes, improve debugging efficiency, and enhance overall user experience. Regular testing, proactive performance monitoring, and robust error-handling mechanisms ensure that mobile applications remain stable, reliable, and efficient. Adopting best practices in crash analysis and debugging will further help in identifying issues early, leading to better app performance and user satisfaction.
Looking for mobile app testing support?
Mundrisoft offers expert guidance and solutions to help businesses optimize app performance, prevent crashes, and streamline debugging processes. Contact us to learn more about our services.